For some reason, I was inspired to write about WordPress Ajax using jQuery. Many WordPress developers are scared about WordPress Ajax including me 🙂 But it is super easy if you understand the basic process of Ajax in WordPress. Just using 3 steps you can complete your Ajax process. First and foremost, the selector, server-side process and jQuery script; then you can display content anywhere on your website.
Now it is time to write code –
HTML Button
First We are going to define a button where we will click for action.
<a href="javascript:void(0)" class="btn btn-primary" id="load-btn">Load Your Action</a>
Server-side process in PHP
In this step, We are going to receive data after the action is complete in jQuery. When we will click on the “Load Your Action” button jQuery will send data to the WordPress function. Now raise a question, how does WordPress identify the actual function? Yes, to identify this, we used a WordPress action hook, this hook’s name is “wp_ajax_” and the next part is “action name” and using this “action name” WordPress can invoke the absolute function. Example: wp_ajax_{your_action} So we can write this action like below –
add_action(‘wp_ajax_your_action’, ‘techidem_func’);
The action name is “your_action”, we will use this inside the jQuery Ajax “action”.
Note: The action name can be anything.
Now, how we can receive the data from jQuery to PHP? We can receive data using the PHP superglobal variable name $_POST. Just follow the below code snippet line no 2.
function techidem_func() {
$recieved_data = $_POST['your_data'];
// Send data to browser console
wp_send_json_success($recieved_data);
}
add_action('wp_ajax_your_action', 'techidem_func'); // private call ( logged-in users )
add_action('wp_ajax_nopriv_your_action', 'techidem_func'); // This action for "No private call" ( non logged-in users )
Get more information about wp_ajax_{$action} and wp_ajax_nopriv_{$action}
Call Ajax using jQuery
<script type="text/javascript">
;(function ($) {
'use strict';
$(document).ready(function () {
$('#load-btn').on('click', function(e) {
$.ajax({
type: "POST",
url: '<?php echo esc_url( admin_url( 'admin-ajax.php' )); ?>',
data: {
action: "your_action", // the action to fire in the PHP server
your_data: {
name : "TechIdem",
url : "https://techidem.com/"
},
},
success: function (response) {
console.log( response );
},
complete: function (response) {
console.log(JSON.parse(response.responseText).data);
},
});
});
});
})(jQuery);
Eventually, we going to understand the jQuery part.
Previously we’ve discussed we will receive data using the PHP $_POST method because we are sending data through the POST method. That’s why we sent a POST request in Ajax.
Now where we are going to send our request?
Yeah, we are sending our request to “admin-ajax.php”. Using “admin-ajax.php” WordPress handle all Ajax requests. Please check line no 12.
Now we are ready to send our data. Using the data object we can send anything to the PHP. For example – action name, nonce, and so on. You can also create multiple JavaScript objects inside the jQuery data.
If the Ajax request is successfully submitted without any error and the status is 200, the success method will execute else error method.
For more details about jQuery Ajax please check the official documentation https://api.jquery.com/jQuery.ajax/
Note: I always love to write JavaScript code under “use strict” mode and it is best practice to avoid unwanted conflicts. And I believe every developer should obey this rule.
Check Result
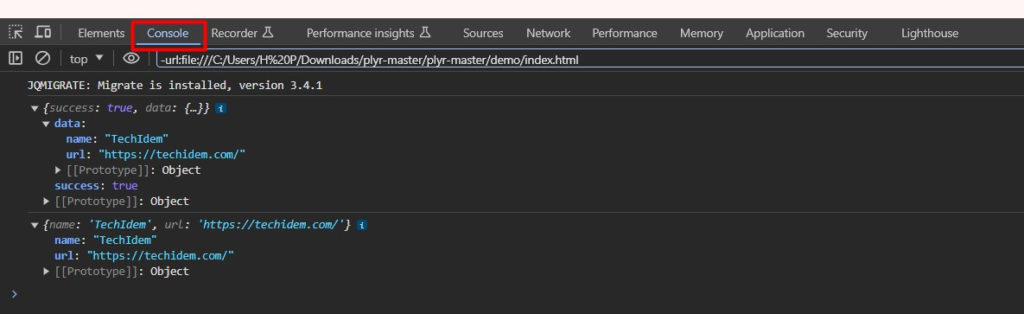